Windows
Setting a Window's Launch Visibility
When ToDesktop renders your application, it goes through a two-step process:
- It creates the native desktop window that surrounds your app.
- It creates a corresponding window view that renders your app.
ToDesktop offers a set of options to help orchestrate when the native desktop window should be made visible:
- Show window: shows the window as soon as it is created. This displays the window faster, but with the downside that the user may see the initial content being rendered.
- Show window when contents loaded: Delays showing the window until the window contents have rendered. This provides the user with a more native rendering experience, but at the cost of the initial window taking longer to appear.
- Hidden: Keeps the window hidden and its contents hidden. This is useful for windows that should stay hidden until some later point. For example, you may want Menubar windows to stay hidden until the tray icon has been clicked.
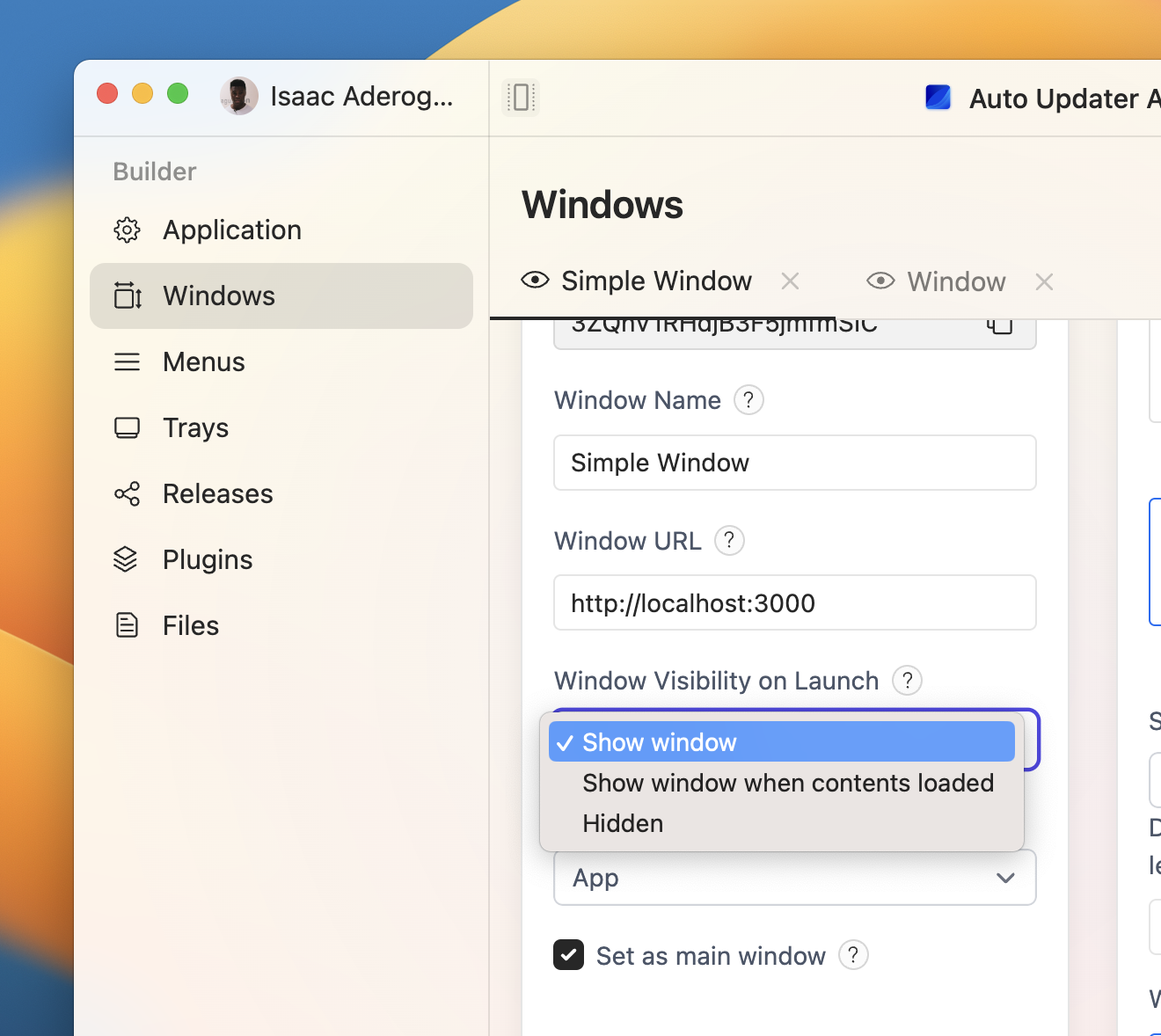
If using the "Show window" option, it's recommended that you choose a window background color that matches the color of your application. We discuss this more in our recipe on changing a window's background color.
You can also change window visibility programmatically with the @todesktop/client-core
NPM library. First install the library in your project:
When creating a window, the show
property will control whether it's made visible on launch:
To only show a window after its contents have been loaded, assign the show
property to false
and create a listener that shows the window only once it's ready.