Releases
Configuring Auto-Updates
ToDesktop automatically updates your desktop app after you release a new version. You can customize this behaviour via the ToDesktop Builder interface or via the @todesktop/client-core
API.
Configuring auto-updates in the UI
Using ToDesktop Builder, navigate to "Releases" via the sidebar. Then scroll down until you see the default auto-updates configuration:
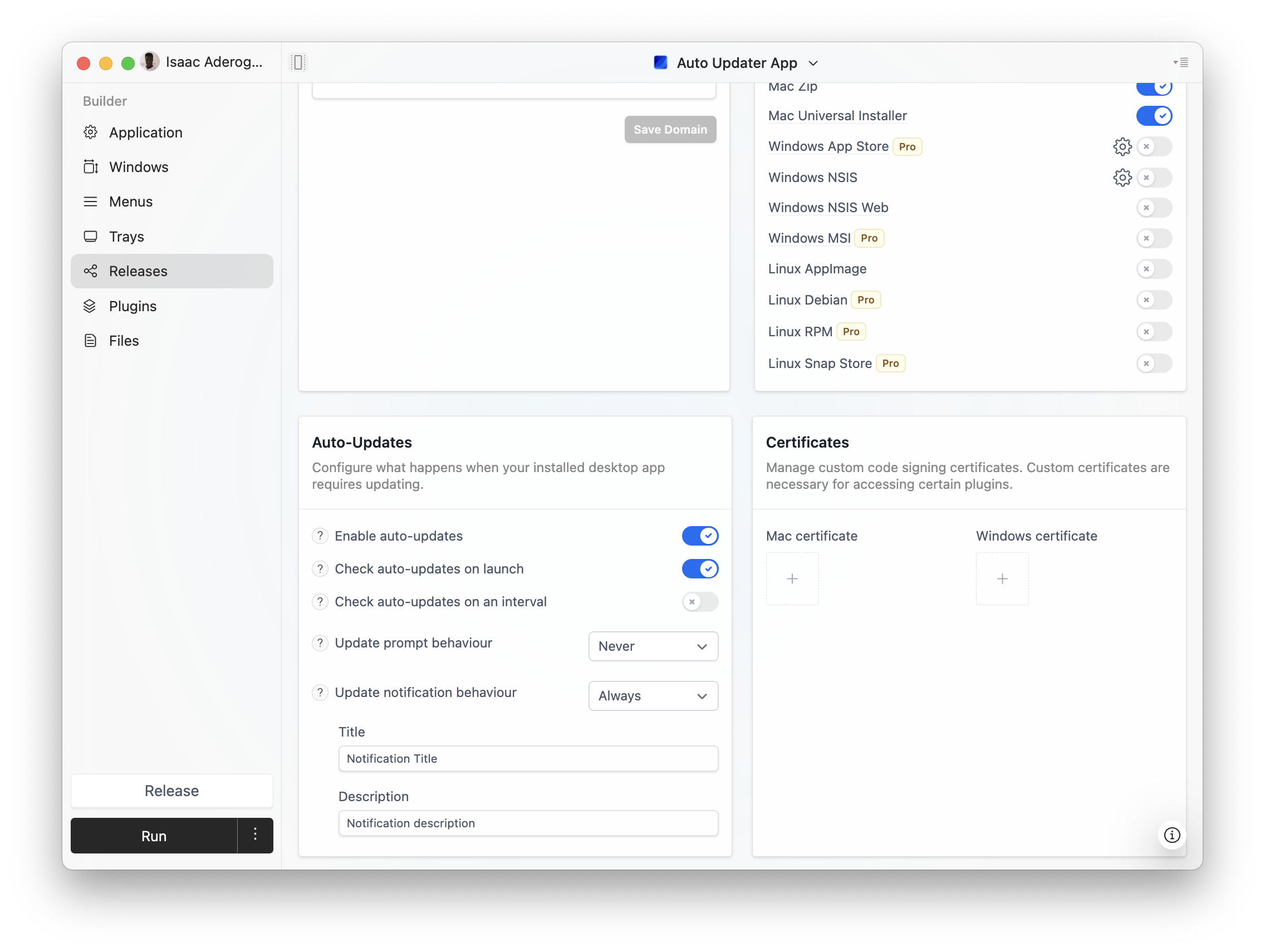
The available options are:
- Enable auto-updates: Whether to enable or disable auto-updates. Only disable auto-updates if you want full programmatic control.
- Check auto-updates on launch: Whether to check for updates when the app is first launched.
- Check auto-updates on an interval: Whether to check for updates on a time interval. Enabling this option will reveal the following sub-options:
- Poll for auto-updates: How often (in minutes) that you'd like to poll for auto-updates.
- Update prompt behaviour: Whether ToDesktop should show a dialog box when a new update is downloaded. "Never" means a user will never be prompted; "Always" means a user will always be prompted; "When in foreground" means a user will only be prompted if the app is currently in focus. Selecting "Always" or "When in foreground" will reveal sub-options for customizing the prompt.
- Update notification behaviour: : Whether ToDesktop should notify a user when a new update is downloaded. "Never" means a user will never be notified; "Always" means a user will always be notified; "When in background" means a user will only be notified if the app is not in focus. Selecting "Always" or "When in background" will reveal sub-options for customizing the notification.
Testing auto-updates locally
To test your auto-update settings locally, ToDesktop offers different modes in which you can run your app. Click the vertical ellipsis icon next to the "Run" button and select "Simulate auto-update events locally".
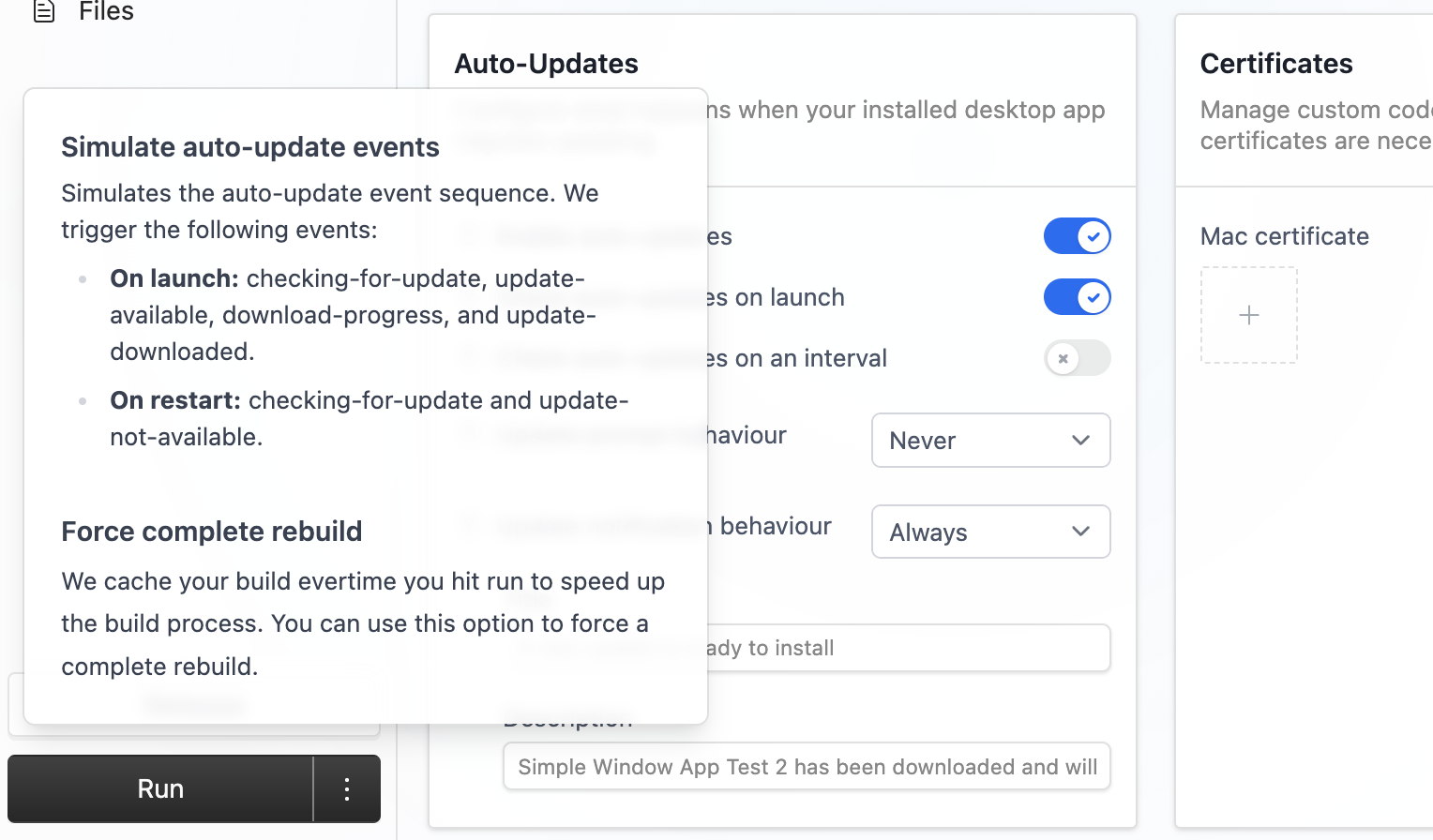
This will run your app with a simulated auto-updater. Behind the scenes, we'll trigger the update-available
and update-not-available
event sequences.
update-available
The update-available
sequence is triggered when the app starts up locally. It will trigger the following events (with a slight delay between each):
checking-for-update
update-available
download-progress
update-downloaded
update-not-available
The update-not-available
sequence will be triggered if you restart the application via the prompt (or programmatically) after an update has been downloaded. This will simulate a situation in which the update has been installed, triggering the following events:
checking-for-update
update-not-available
Disabling auto-updates via the UI will also disable the simulated update-available
and update-not-available
event sequences that occur on launch. You will still be able to trigger these sequences programmatically.
Managing auto-updates programmatically
Auto-updates can also be managed programmatically via the @todesktop/client-core
API. This API can be used to either check for updates, install updates, or listen to underlying events.
Checking for updates
You can check for updates programmatically by invoking todesktopUpdater.checkForUpdates
. If an update is available, it will be downloaded before the function return value is resolved.
In these situations, it may also be helpful to set disableUpdateReadyAction
to true
. This allows you to explicitly control the prompt and notification behaviour, without worrying about interference from the settings that you've configured in the ToDesktop Builder UI.
If disableUpdateReadyAction
is set to false
(or not defined), ToDesktop will default to what you've specified for "Update prompt behaviour" and "Update notification behaviour" in ToDesktop Builder:
This approach is useful if you want to periodically check for updates, but don't want to wire up the code for conditionally displaying a prompt and notification.
Installing updates
Updates can be installed by invoking todesktopUpdater.restartAndInstall
. It's important that this function is only called if there's an available update. For example:
Listening to events
Finally, you can listen to auto-update events that are emitted from ToDesktop by using todesktopUpdater.on
:
The available events are:
error
before-quit-for-update
checking-for-update
download-progress
update-available
update-not-available
update-downloaded
The events listed above can also be trigger if you're running in simulation mode. This means that you can test auto-update behaviour from the safety of your local development environment.