Files
How to Interact with the File System using ToDesktop Plugins
ToDesktop plugins are a great way to add native functionality to your desktop application. ToDesktop's file system (FS) plugin enables you to securely read and write files to the local file system.
ToDesktop Builder Configuration
Using the ToDesktop Builder desktop application, navigate to the "Plugins" menu and look for the "File System" plugin. Once located, click "Explore" to navigate to the plugin view and proceed to install the plugin.
Once installed, you should see a suite of configuration options for managing the plugin's behaviour:
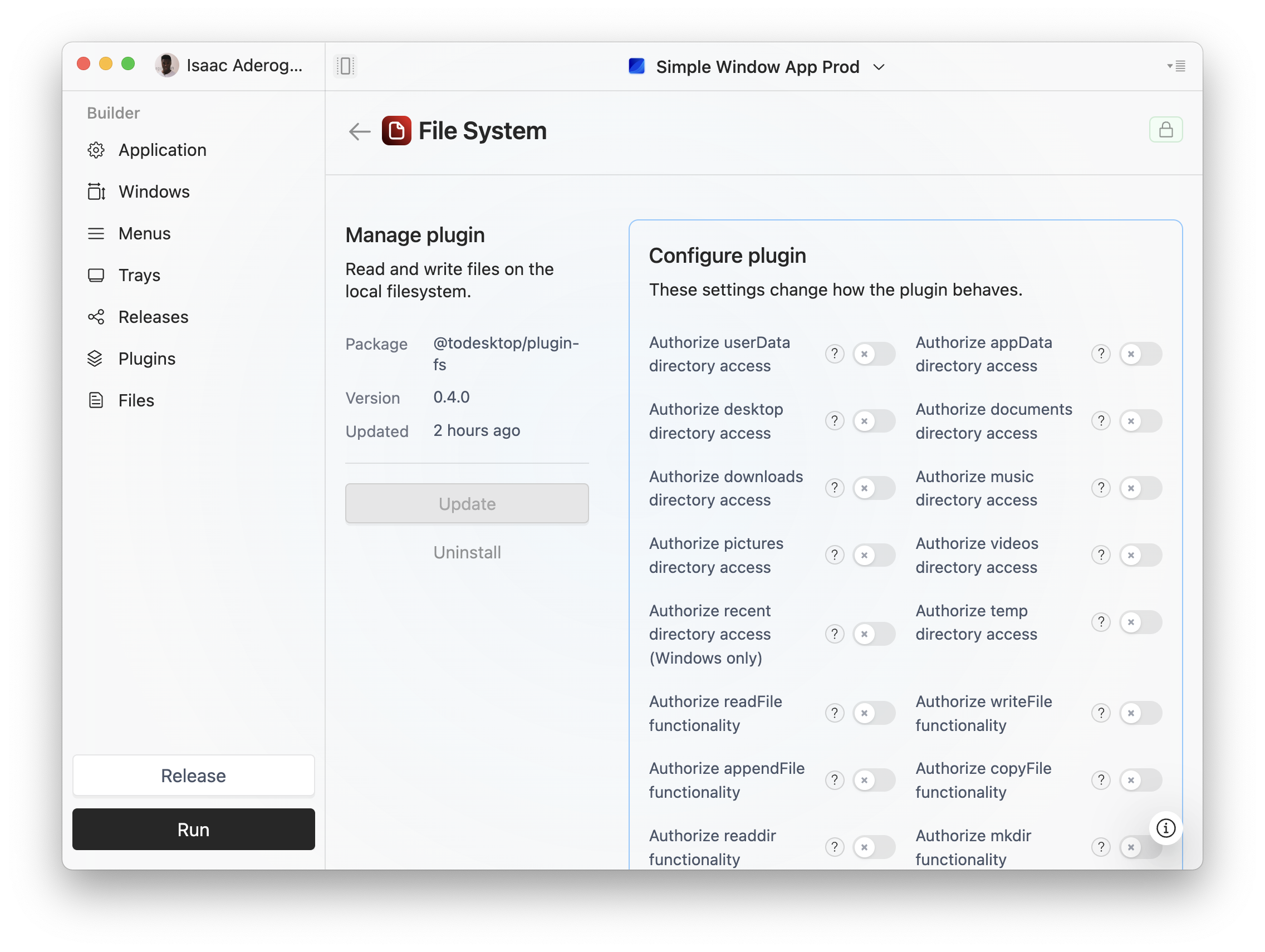
These options allow you to fine-tune the behaviour of your plugin. All of these options are disabled by default, meaning that the FS plugin will prohibit all functionality. For now, we'll want to support reading files from the downloads directory. To authorize this, check the following configuration options:
- Authorize downloads directory access.
- Authorize readdir functionality.
You should only authorize the specific behaviour and folder access that you need. By authorizing functionality, we're allowing it to be accessed by your client-side application.
Granting excessive permissions can expose sensitive user data and widen the attack surface for potential vulnerabilities. It's essential to adopt a principle of least privilege to safeguard user data and reduce the risk of exploits.
Client App Usage
To use the FS plugin from your client-side application, install the following package:
Reading the downloads
directory is then as simple as the following:
Note that the readdir
function will throw an error if passed a directory that doesn't correspond to what you have authorized. Similarly, trying to use a different function, such as writeFile
, will also throw an error if it has not been explicitly authorized:
To progammatically see which permissions have been granted, you can use the getPluginContext
function to read your configuration choices:
Release Considerations
When attempting to release an application with the fs
plugin enabled, you may see this warning:
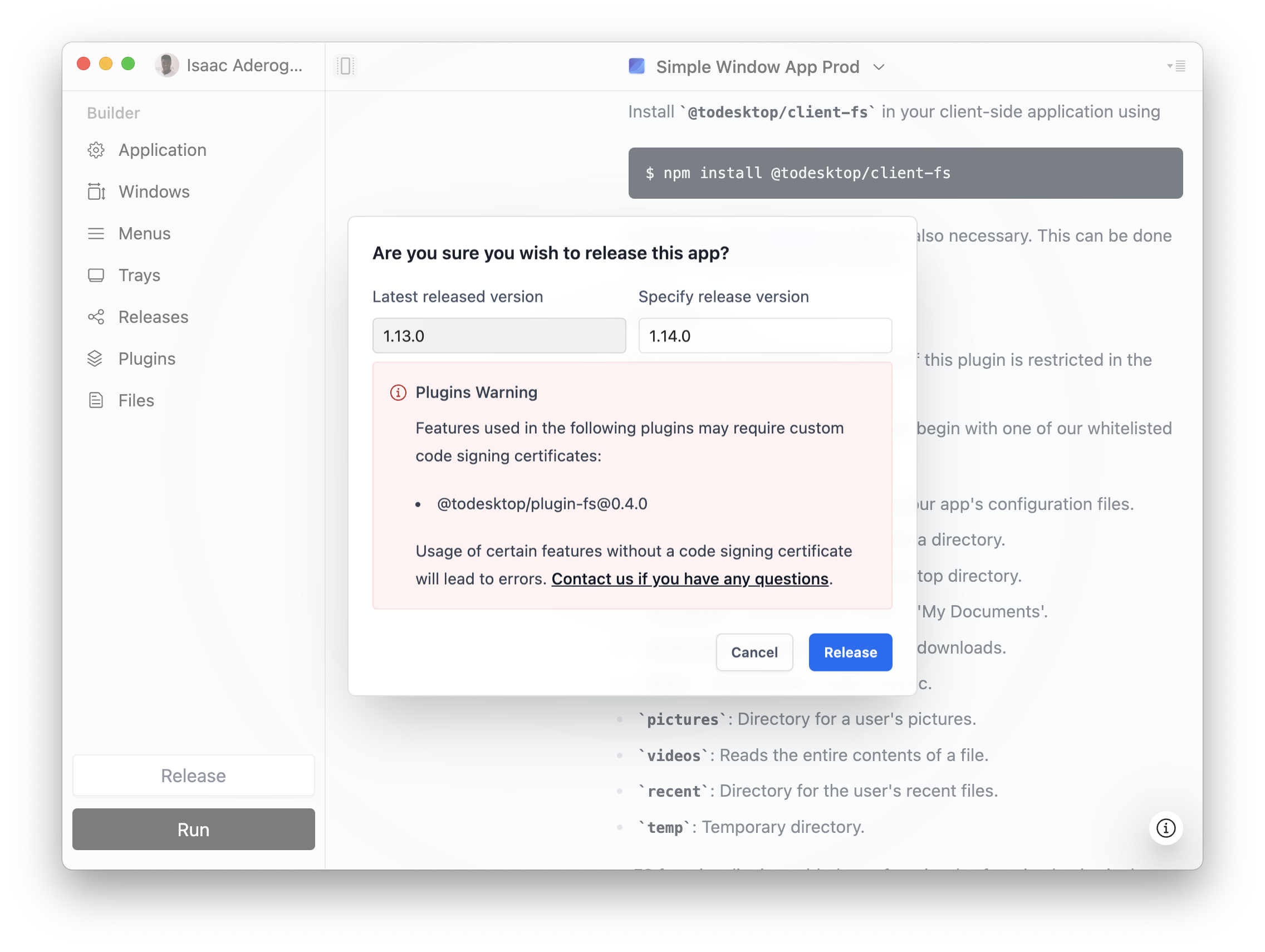
This warning occurs because certain plugins, like the FS plugin, require custom code signing certificates in order to be used in production. It's important to ensure that you have the correct code signing certificates, otherwise the plugin will throw an error when any of its functionality is invoked at runtime.
To set up a certificate, you will need to navigate to "Releases" via the sidebar and then scroll down to the "Certificates" card:
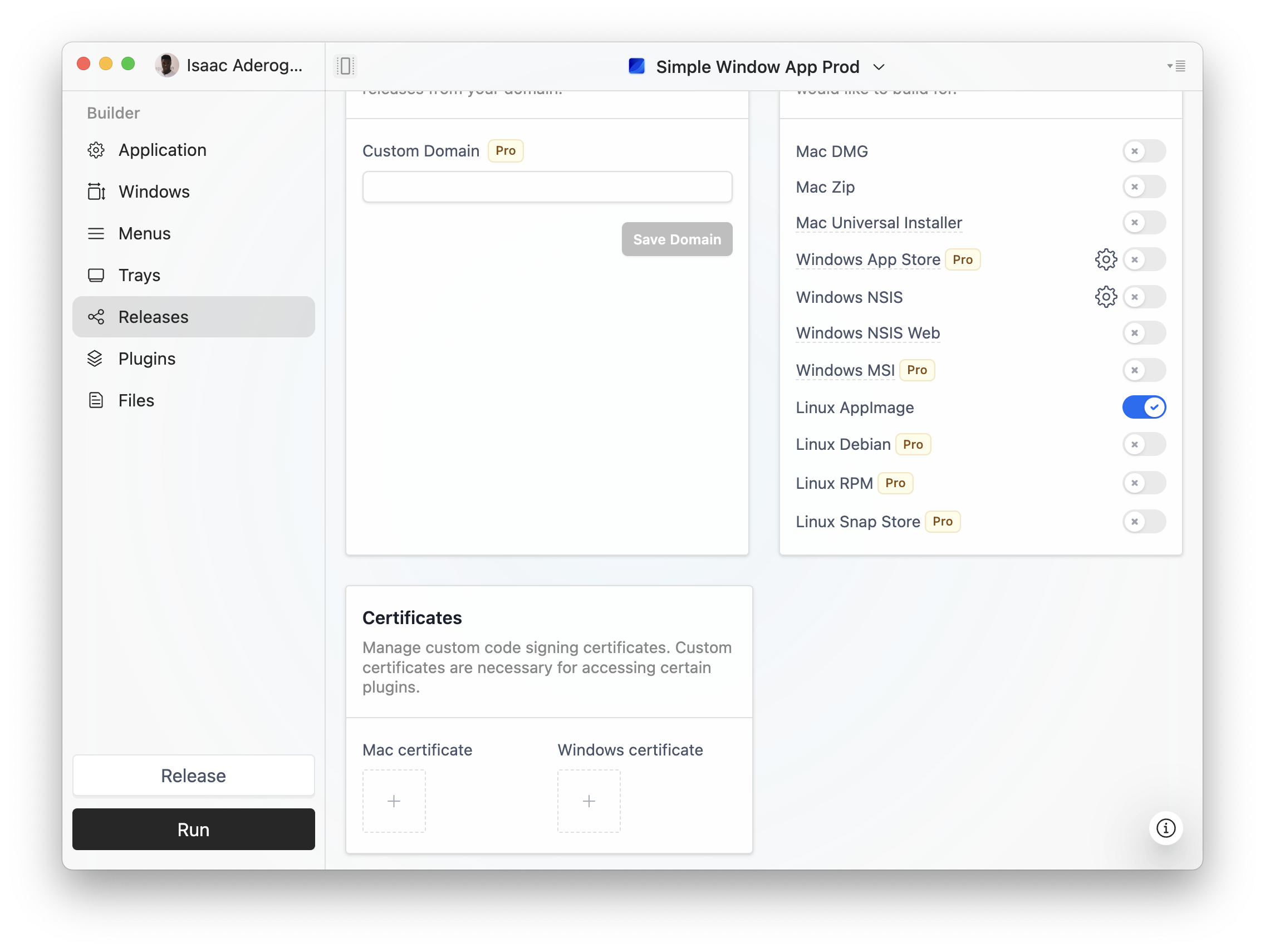
Clicking the add button under the Mac or Windows certificate will navigate you to the web application where you can proceed to upload your custom code signing certificates. This can be a complicated process, so feel free to contact us at [email protected] if you would like to learn more.