Windows
Handle window.open by creating dynamic rules
Windows inherit the rules of their parent window
When you create a new window with window.open
it inherits the rules and styles of the window that created it. Sometimes this is not what you want. For example, you might want to open a new window that is not resizable or maximizable. Or perhaps the parent window has no title bar, but you want the new window to have a title bar.
For this reason, you can use the webContents.setWindowOpenRules
method to set rules for how new windows should be opened.
setWindowOpenRules
The setWindowOpenRules
method takes an object with the following properties:
ref
- The web contents reference of the window that will be used to open new windows. If not provided, the current window will be used.rules
- An array of rules to apply when opening new windows.rules[].regex
- A regular expression that will be used to match the URL of the new window.rules[].options.action
- The action to take when the URL matches the regular expression. Can be one of the following:allow
- Allow the window to open.deny
- Deny the window from opening.openInBrowser
- Open the URL in the user's default web browser.
rules[].options.overrideBrowserWindowOptions
- An object containing options that will be used to override the default browser window options. We support most of Electron'sBrowserWindowConstructorOptions
.
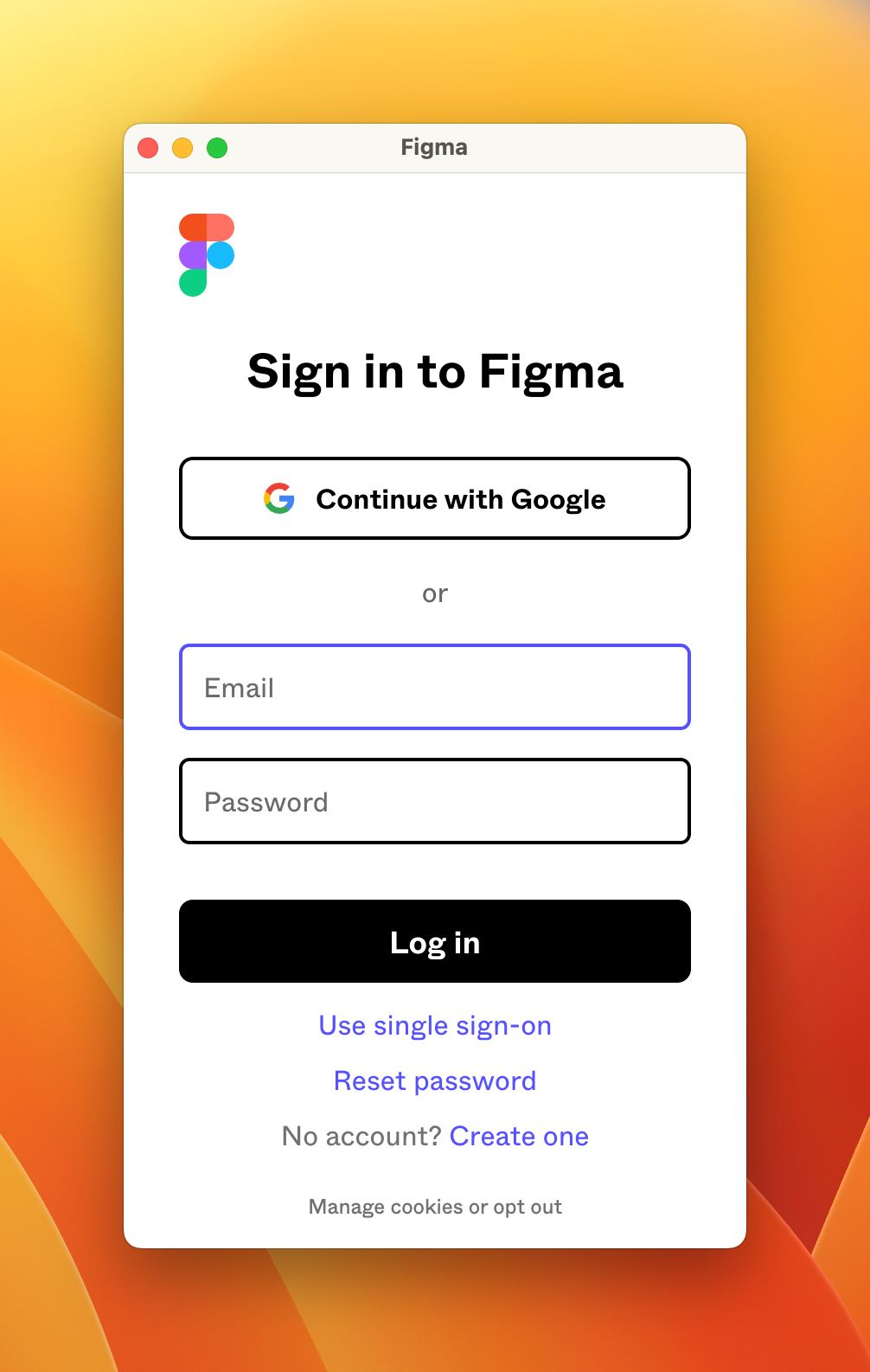